The Java Collection Framework provides a comprehensive set of interfaces, classes, and algorithms to manipulate and store groups of objects efficiently. This versatile framework forms the backbone of Java programming, offering a multitude of data structures and algorithms. In this detailed guide, we'll explore the nuances of the Collection Framework, its core components, implementations, and usage scenarios.
Understanding Collections
1. Collections Hierarchy
The Collection Framework includes interfaces such as `Collection`, `List`, `Set`, and `Map`, among others. These interfaces serve as blueprints for different data structures, defining common behaviours and operations.
2. Core Interfaces
2.1 List Interface
ArrayList: A dynamically resizing array implementation.
LinkedList: Doubly linked list implementation, suitable for frequent insertion/deletion operations.
Vector: Similar to ArrayList but synchronized.
2.2 Set Interface
HashSet: Implements the Set interface using a hash table for storage.
TreeSet: Implements a set stored in a tree structure, providing ordered elements.
2.3 Map Interface
HashMap: Stores key-value pairs using a hash table.
TreeMap: Implements a Map stored in a tree structure, providing ordered keys.
3. Iterators and Iterables
Iterators allow sequential access to elements in a collection. The `Iterable` interface provides the ability to iterate through collections using the enhanced forloop.
Implementations and Usage
1. Lists
ArrayList
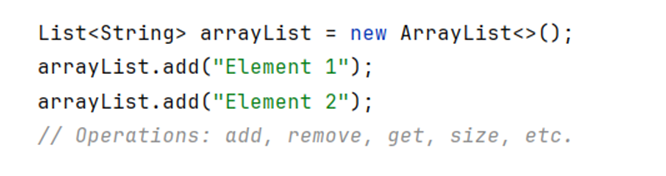
LinkedList
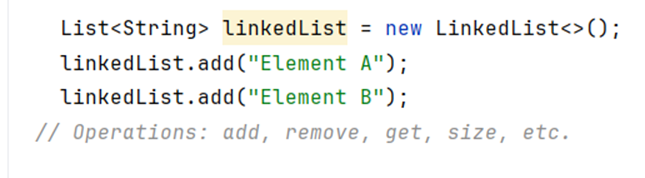
2. Sets
HashSet
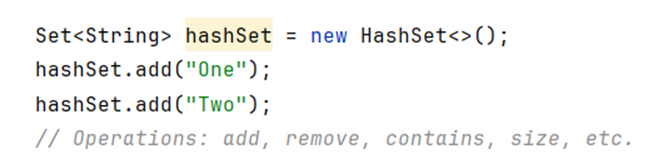
TreeSet
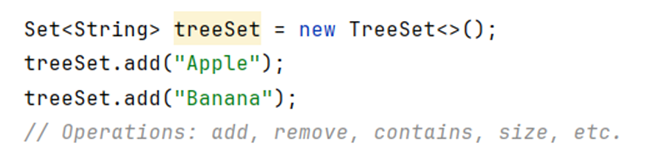
3. Maps
HashMap

TreeMap
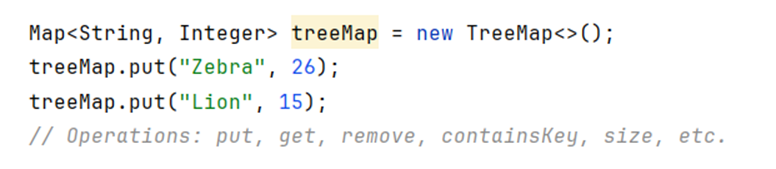
Best Practices and Tips
1. Choose the Right Collection
Select the appropriate collection based on your requirements whether it's maintaining order, ensuring uniqueness, or optimizing search and retrieval operations.
2. Generics Usage
Utilize generics to specify the type of elements within collections, ensuring type safety and avoiding runtime errors.
3. Understand Time Complexity
Different collection implementations offer different time complexities for operations. Be aware of these complexities to make informed decisions based on performance needs.
4. Favor Interfaces Over Implementations
Program against interfaces (`List`, `Set`, `Map`) rather than specific implementations for flexibility and easier maintenance.
Conclusion
The Java Collection Framework is a powerful arsenal for Java developers, offering a plethora of data structures and algorithms to manage and manipulate collections of objects efficiently. Understanding the nuances of each interface, and their implementations, and choosing the right data structure for specific tasks is key to writing efficient and robust Java programs.
In this blog, we've explored the core components, implementations, best practices, and usage scenarios of the Java Collection Framework. Embrace these concepts to empower your Java programming skills and build high-performing applications efficiently.
Comments