A Java enumeration is a class type. Although we do not need to instantiate an enum with new, it has the same features as other classes. This fact makes Java enumeration an extremely useful tool. You may give them constructors, instance variables, methods, and even interfaces, just like you would with classes.
Declaration of enum in Java
Enum declarations are allowed both inside and outside of classes, but not within methods.
1. Declaration outside the class
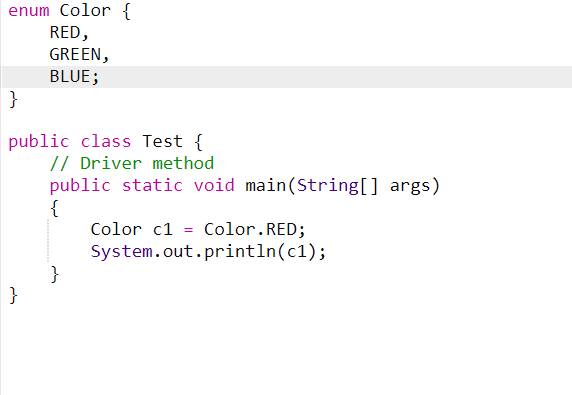
Output:

2. Declaration inside a class
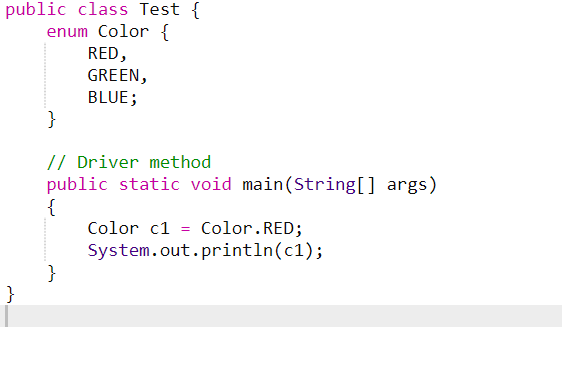
Output:
Properties of Enum in Java
Enum follows certain properties, which are listed below:
Each enum is internally implemented using Class.
Every enum constant represents an enum-type object.
An enum type can be used as an argument in switch statements.
Every enum constant is inherently public static and final. Because it is static, we can access it using the enum Name. Because it is final, we cannot build child enums.
We can declare the main() method within the enum. As a result, we can invoke the enum directly from the Command Prompt.
Methods of Java Enum Class
There are some predefined methods in enum classes that are readily available for use.
1. Java Enum ordinal()
The ordinal() method returns the position of an enum constant. For example,
ordinal(SMALL)
// returns 0
2. Enum compareTo()
The compareTo() method compares the enum constants based on their ordinal value. For example,
Size.SMALL.compareTo(Size.MEDIUM)
// returns ordinal(SMALL) - ordinal(MEDIUM)
3. Enum toString()
The toString() method returns the string representation of the enum constants. For example,
SMALL.toString()
// returns "SMALL"
4. Enum name()
The name() method returns the defined name of an enum constant in string form. The returned value from the name() method is final. For example,
name(SMALL)
// returns "SMALL"
5. Java Enum valueOf()
The valueOf() method takes a string and returns an enum constant having the same string name. For example,
Size.valueOf("SMALL")
// returns constant SMALL.
6. Enum values()
The values() method returns an array of enum type containing all the enum constants. For example,
Size[] enumArray = Size.value();
Comments