In Java, an exception is an event that disrupts the normal flow of the program's instructions during execution. When an error or exceptional condition occurs, Java generates an exception object and throws it. This exception object contains information about the error, such as its type and message, allowing the programmer to handle the error gracefully. Here are some of the commonly encountered exceptions in Java along with detailed explanations:
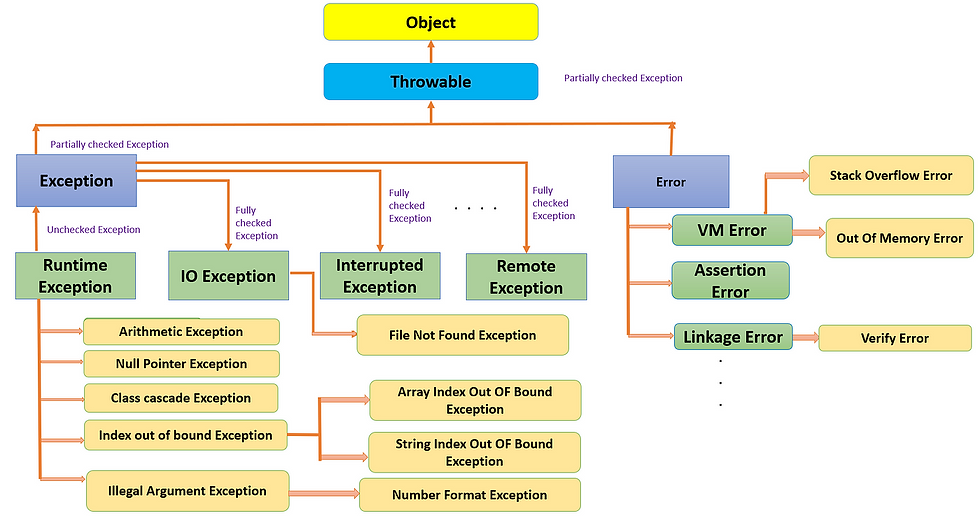
· NullPointerException:
This exception occurs when you try to access or manipulate an object that has a null value. In Java, every object must be initialized before use. If an object reference is not initialized or set to null, trying to access its members or methods will result in a NullPointerException.
Example:
String str = null;
System.out.println(str.length()); // This will throw a NullPointerException
· ArrayIndexOutOfBoundsException:
This exception occurs when you try to access an index that is outside the bounds of an array. In Java, array indices start from 0 to length-1. If you try to access an index beyond these bounds, Java will throw an ArrayIndexOutOfBoundsException.
Example:
int[] arr = {1, 2, 3};
System.out.println(arr[3]); // This will throw an ArrayIndexOutOfBoundsException
· ArithmeticException:
This exception occurs during arithmetic operations, primarily division by zero. In Java, dividing an integer by zero results in an ArithmeticException.
Example:
int result = 10 / 0; // This will throw an ArithmeticException
· ClassCastException:
This exception occurs when you try to cast an object to a class type that it is not an instance of. Java supports casting between compatible types, but if you attempt to cast between incompatible types, a ClassCastException will be thrown.
Example:
Object obj = new Integer(10);
String str = (String) obj; // This will throw a ClassCastException
· FileNotFoundException:
This exception occurs when attempting to access a file that does not exist. It is a subclass of IOException and is thrown by classes like FileInputStream, FileReader, etc., when the specified file path cannot be found.
Example:
try {
FileInputStream fis = new FileInputStream("file.txt");
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
· IOException:
This exception is a general class of exceptions produced during input/output operations. It's often thrown when there is an error while reading from or writing to a file, socket, or stream.
Example:
try {
FileReader fileReader = new FileReader("file.txt");
char[] buffer = new char[1024];
fileReader.read(buffer);
} catch (IOException e) {
System.out.println("IOException occurred: " + e.getMessage());
}
· NumberFormatException:
This exception occurs when you try to convert a string to a numeric type, but the string does not contain a valid numeric value. For example, trying to parse a string containing letters into an integer will result in a NumberFormatException.
Example:
String str = "abc";
int num = Integer.parseInt(str); // This will throw a NumberFormatException
· OutOfMemoryError:
This error occurs when the Java Virtual Machine (JVM) runs out of memory. It usually happens when an application attempts to allocate more memory than the JVM can provide. Common causes include memory leaks or attempting to load very large data sets into memory.
Example:
List<Integer> list = new ArrayList<>();
while (true) {
list.add(10); // This will eventually throw an OutOfMemoryError
}
· StackOverflowError:
This error occurs when the call stack of a program exceeds its maximum size. It usually happens due to excessive recursion, where a method calls itself repeatedly without reaching a base case.
Example:
public void recursiveMethod() {
recursiveMethod();
}
recursiveMethod(); // This will throw a StackOverflowError
Comments