Exploring the Java Collection Framework: A Comprehensive Overview
- patilrohina
- Mar 14, 2024
- 3 min read
In the world of Java programming, the Collection Framework stands as a cornerstone, providing a rich set of data structures and algorithms for storing, manipulating, and accessing collections of objects. In this comprehensive blog post, we'll embark on a journey through the Java Collection Framework, unraveling its components, usage, benefits, and best practices.
Understanding the Collection Framework:
The Java Collection Framework is a unified architecture for representing and manipulating collections of objects. It consists of interfaces, implementations, and algorithms that enable developers to work with collections in a standardized and efficient manner. The key interfaces in the Collection Framework include Collection, List, Set, Map, and their respective implementations.
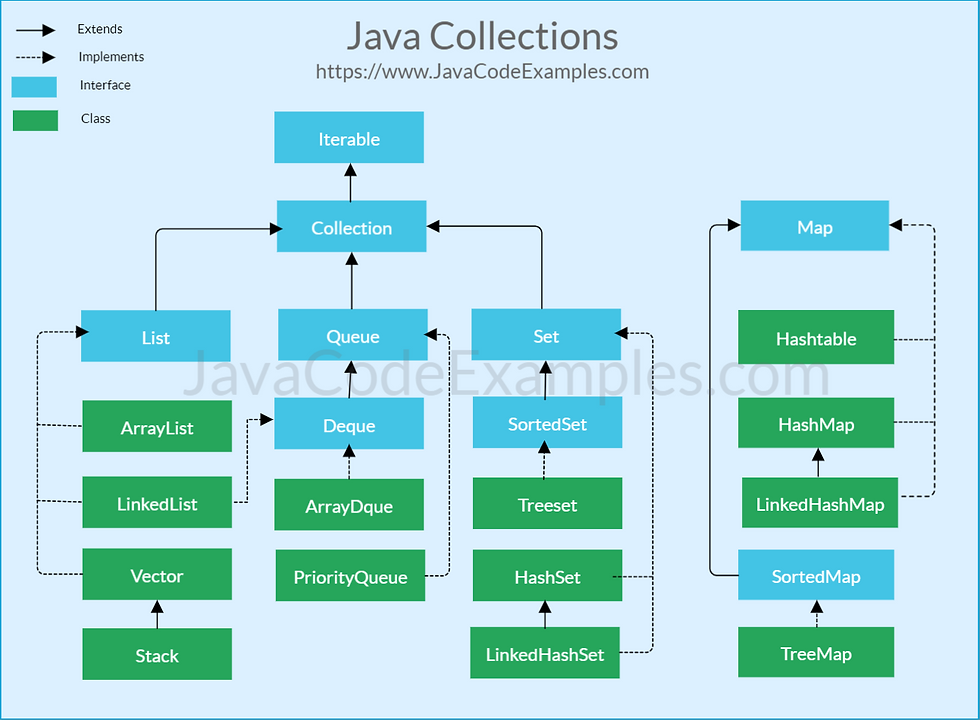
Key Components:
Interfaces:
Collection: The root interface in the Collection Framework hierarchy, representing a group of objects.
List: An ordered collection that allows duplicate elements.
Set: A collection that does not allow duplicate elements.
Map: A mapping between keys and values, where each key is associated with a single value.
Implementations:
ArrayList: A dynamic array implementation of the List interface.
LinkedList: A doubly linked list implementation of the List interface.
HashSet: A hash table-based implementation of the Set interface.
TreeSet: A red-black tree-based implementation of the Set interface that maintains elements in sorted order.
HashMap: A hash table-based implementation of the Map interface.
TreeMap: A red-black tree-based implementation of the Map interface that maintains key-value pairs in sorted order.
Algorithms:
Sorting: Collection Framework provides sorting algorithms such as Collections.sort() for sorting collections.
Searching: Algorithms like binary search (Collections.binarySearch()) are available for searching elements in sorted collections.
Usage and Benefits:
Ease of Use: The Collection Framework provides a high-level abstraction for working with collections, simplifying the development process and improving code readability.
Performance: The implementations in the Collection Framework are optimized for performance, providing efficient data structures and algorithms for common operations such as insertion, deletion, and retrieval.
Flexibility: The framework offers a wide range of data structures and algorithms, allowing developers to choose the most suitable collection type for their specific use case.
Interoperability: Collections in the framework are interoperable, meaning they can be easily converted from one type to another using utility methods provided by the framework.
Standardization: The Collection Framework follows a standard set of interfaces and conventions, making it easier for developers to understand and use collections across different libraries and frameworks.
Best Practices:
Choose the Right Collection Type: Select the appropriate collection type based on the requirements of your application, considering factors such as performance, ordering, and uniqueness constraints.
Use Generics: Take advantage of generics to ensure type safety and avoid runtime errors when working with collections.
Minimize Mutability: Prefer immutable collections (Collections.unmodifiableXXX()) or make collections immutable whenever possible to avoid unintended modifications.
Avoid Raw Types: Avoid using raw types (e.g., ArrayList without specifying a type parameter) as they bypass compile-time type checks and can lead to runtime errors.
Use Enhanced for Loop: Utilize the enhanced for loop (for-each) for iterating over collections, as it provides a cleaner and more concise syntax.
Conclusion:
The Java Collection Framework serves as a fundamental building block for Java developers, providing a versatile and efficient framework for working with collections of objects. By understanding its components, usage patterns, and best practices, developers can leverage the power of the Collection Framework to build robust, scalable, and maintainable Java applications. Whether you're working on a small project or a large-scale enterprise application, mastering the Collection Framework is essential for writing clean, efficient, and elegant code. So, dive into the world of collections and unlock the full potential of Java programming.
Commentaires