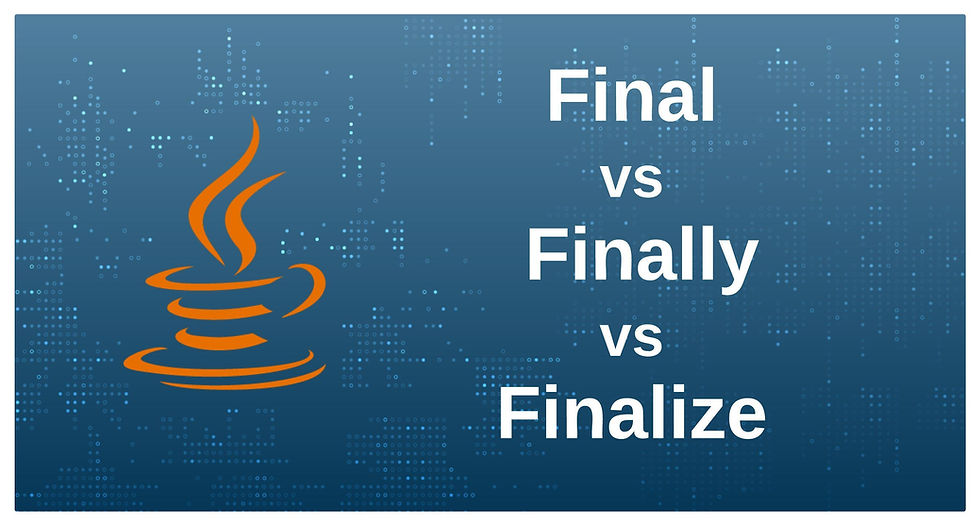
Introduction
In the realm of Java programming, three pivotal keywords stand out — 'final', 'finally', and 'finalize'. While they might seem similar, their roles diverge significantly within the Java language. This blog post aims to dissect each keyword, elaborating their distinctions through code examples, thereby facilitating clearer comprehension of its functionalities.
final Keyword:
The 'final' keyword is employed to define variables, methods, and classes that retain their values throughout the code execution and cannot be modified thereafter.
Final Variable:
In Java, a final variable is one that's assigned a value only once. Once initialized, its value remains unchanged for the duration of the program's execution. These variables, essentially constants, are usually declared using the 'final' keyword.
Example:
public class FinalVariable {
public static void main(String[] args) {
final int a = 11;
//a = 33; This will result in compilation error as 'a' is declared final
System.out.println("The value of a is: " + a);
}
}
Final Method:
In Java, a final method is one that's incapable of being overridden by subclasses. When a method is marked as final within a superclass, it signifies that subclasses are restricted from altering its implementation. This guarantees that the behavior initially defined in the superclass remains consistent across all subclasses.
Example:
class Parent {
final void finalMethod() {
System.out.println("final Method of Parent class");
}
}
class Child extends Parent {
// overriding the finalMethod will result in a compilation error
/* @Override
void finalMethod() {
System.out.println("This method cannot be overridden.");
} */
}
public class Main {
public static void main(String[] args) {
Parent p = new Parent();
p.finalMethod(); // Output: final Method of Parent class
Child c = new Child();
c.finalMethod(); // Output: final Method of Parent class
}
}
Final Class:
In Java, a final class is one that cannot be extended or subclassed by other classes. By declaring a class as final, it signifies that its implementation is concluded and cannot be altered or extended any further.
Example:
final class FinalClassDemo {
void display() {
System.out.println("In Final Class.");
}
}
// Attempt to subclass a final class will result in a compilation error
/*class SubClass extends FinalClassDemo {
// Compilation error: Cannot inherit from final FinalClassDemo
}*/
Finally:
The finally block is certain to execute upon the completion of the try block, ensuring its execution even if an unexpected exception arises. However, the significance of finally extends beyond handling exception, it serves as a safeguard to ensure that cleanup code isn't accidentally overlooked due to return, continue, or break statements. It's regarded as a best practice to incorporate cleanup code within a finally block, even in situations where exceptions are not anticipated.
Example:
public class FinallyDemo {
public static void main(String[] args) {
try {
int res = 11 / 0; // Division by zero, will throw an ArithmeticException
System.out.println("Result: " + res); // This line will not be executed
} catch (ArithmeticException e) {
System.out.println("Error: Division by zero!");
} finally {
System.out.println("Finally block executed.");
}
}
}
The catch block will catch the exception and prints the error message. No matter if an exception occurs or not, the finally block will always be executed, printing the message "Finally block executed."
Finalize:
In Java, the finalize() method, which is part of the Object class, gets invoked by the garbage collector prior to reclaiming the memory held by an object that's no longer accessible or required by the program. Its role is to enable an object to execute cleanup tasks before it undergoes garbage collection.
Example:
public class FinalizeDemo {
protected void finalize() {
System.out.println("Finalize method called.");
// Perform cleanup tasks here
}
public static void main(String[] args) {
MyClass obj = new MyClass();
// Set obj to null to make it eligible for garbage collection
obj = null;
// Trigger garbage collection
System.gc();
}
}
Invoking the gc method implies that the Java Virtual Machine will make an attempt to reclaim memory occupied by unused objects, aiming to swiftly free up the memory for potential reuse.
Conclusion
To summarize, the 'final' keyword is employed to establish constants or immutable elements, 'finally' is utilized in exception handling to ensure the execution of code irrespective of any exceptions thrown, and 'finalize' is a specialized method invoked by the garbage collector before reclaiming an object.
Thanks for taking the time out to read this blog post.
Happy Learning !!
Comments