Exception handling is a crucial aspect of software development and is used to handle unexpected errors or conditions in a program. for understanding the term exception handling in java let's first understand what is exception exactly is, Exception is an event that occurs during the execution of the program that disrupts the normal flow of the program. For our program to be run successfully it is required to solve any exception occurred in our program. If you don't deal with an exception when it occurs, the program will end suddenly.
Exception occur for various reasons like
A user has entered an invalid data
File not found
A network connection has been lost in the middle of communications
The JVM has run out of a memory
Why is Exception Handling Important in Java?
Exception handling is important in Java for several reasons. Firstly, it helps to ensure that the program continues to run, even in the face of unexpected errors or conditions. Secondly, it helps to prevent the program from crashing or producing incorrect results. Thirdly, it makes it easier to debug and maintain the code, as exceptions can be handled and resolved in a structured way.
How to Implement Exception Handling in Java
Implementing exception handling in Java involves the following steps:
Identify the exceptions that may occur in the program
Use try-catch blocks to catch exceptions
Use the throw statement to throw exceptions
Use the finally block to specify code that will be executed regardless of whether an exception is thrown or caught
The try-catch block is the cornerstone of exception handling in Java. The try block contains the code that might throw an exception, and the catch block contains the code that will handle the exception. The catch block is used to catch exceptions and to handle them in a controlled manner.
Here is a simple example of exception using a try-catch block in Java:
try {
int result = 50/0;
}catch(ArithmeticException e){
System.out.println("ArithmeticException caught: " + e.getMessage());
}
In the above example, the try block contains the code that might throw an exception (dividing by zero), and the catch block contains the code that will handle the exception (printing an error message).
The throw statement is used to throw an exception in Java. This statement can be used to throw a custom exception, or to throw an exception of a specific type. Here is an example of using the throw statement in Java:
What is the Exception Hierarchy in Java?
The exception hierarchy in Java is a hierarchy of exception classes that are used to categorize and handle different types of exceptions in a consistent and organized manner. The exception hierarchy in Java is centered around the Throwable class, which is the root class of all exceptions and errors in Java. The Throwable class has two direct subclasses: Error and Exception. The Error class represents serious problems that are beyond the control of the program, such as hardware errors or memory overflow. The Exception class represents exceptions that can be handled by the program, such as runtime errors or invalid data input.
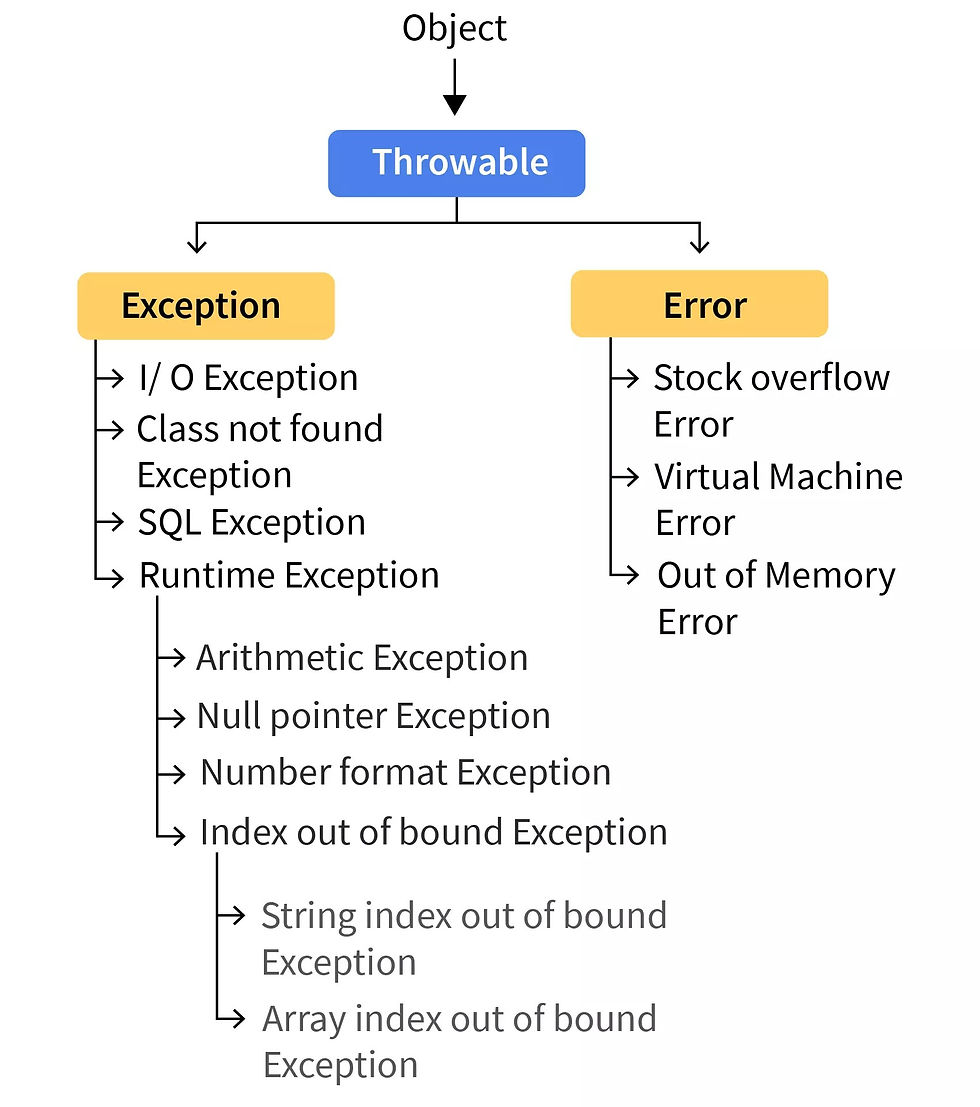
To choose the appropriate exception class to use for an exception, you should consider the type of exception and the severity of the exception. For example, if you are dealing with a runtime error, you may choose to use the RuntimeException class. If you are dealing with an invalid data input exception, you may choose to use the IllegalArgumentException class.
What are the different types of Exceptions in Java ?
There are two types of exceptions in Java: checked exceptions and unchecked exceptions. Understanding the difference between these two types of exceptions is essential for writing robust and reliable Java programs.
Checked Exceptions:
Checked exceptions are exceptions that the Java compiler forces you to catch or declare.
Examples of checked exceptions include FileNotFoundException, IOException, and SQLException.
Checked exceptions represent conditions that are considered recoverable and that the programmer must take care of.
Checked exceptions must be either caught with a try-catch block or declared in the method signature using the throws keyword.
Unchecked Exceptions:
Unchecked exceptions are exceptions that the Java compiler does not force you to catch or declare.
Examples of unchecked exceptions include NullPointerException, ArrayIndexOutOfBoundsException, and IllegalArgumentException.
Unchecked exceptions represent conditions that are considered unexpected and that the programmer is not required to handle.
Unchecked exceptions are typically runtime errors and are thrown automatically by the Java runtime system.
In general, checked exceptions are used for exceptional conditions that the programmer can reasonably be expected to anticipate and handle, while unchecked exceptions are used for exceptional conditions that are beyond the control of the program. When designing your Java programs, it is important to understand the difference between checked and unchecked exceptions and to use them appropriately. Kindly refer to the image below to understand more about difference between checked and unchecked exception

What are Exception keywords in Java ?
The following are the three main keywords in Java for handling exceptions:
Try: The try block is used to enclose the code that may cause an exception. If an exception occurs within the try block, the exception is caught and handled by the catch block.
Catch: The catch block is used to handle the exceptions thrown by the try block. The catch block is executed only if an exception occurs in the try block. You can have multiple catch blocks with different exception types to handle different exceptions in the try block.
Finally: The finally block is used to execute a block of code regardless of whether an exception occurs or not. It is typically used to release resources, such as file handles or database connections, that have been acquired in the try block.
In addition to these keywords, Java also provides the following keywords to handle exceptions:
Throw: The throw keyword is used to explicitly throw an exception. This can be used to throw a custom exception or any other exception type in the Java standard library.
Throws: The throws keyword is used in the method signature to indicate that a method may throw an exception. It is used to propagate the exception to the caller of the method.
Here is a simple example of each keyword of Exception in Java:
Try block
try{
//Doubtful Statements.
}
Catch block
try{
//Doubtful Statements}
catch(Exception e)
{
}
Finally block
try{
//Doubtful Statements}
catch(Exception e)
{
}
finally{
//Close resources
}
Throw keyword
public class Main {
static void checkAge(int age) {
if (age < 18) {
throw new ArithmeticException("Access denied - You must be at least 18 years old.");
} else {
System.out.println("Access granted - You are old enough!");
}
}
Throws keyword
public class Main {
static void checkAge(int age) throws ArithmeticException {
if (age < 18) {
throw new ArithmeticException("Access denied - You must be at least 18 years old.");
} else {
System.out.println("Access granted - You are old enough!");
}
}
By using the exception keywords, you can handle exceptions in a controlled and organized manner. This helps you to write more robust and maintainable Java code that can handle errors and unexpected events gracefully.
Conclusion
Programmers can deal with undesirable circumstances by using exception handling.
By extending the Exception class, Java offers the ability to specify and use custom exceptions.
Exception handling makes the program efficient in terms of handling errors/exceptions and user-friendly, as user can understand the exceptions easily.
Java uses exception handling very efficiently, basically with five keywords try, catch, finally, throw, and throws.
Comentários