Hibernate Lifecycle
- Jaypalsinh Ramlavat
- Feb 12, 2024
- 2 min read
Hibernate Entities
Let's take a look at some fundamental components of the hibernate lifecycle before diving right into the various stages.
Using Hibernate requires the use of Plain Java Objects (POJO). If there are no hibernate-specific annotations present, the POJO classes cannot be identified in their raw form.
But Hibernate will be able to recognize and interact with the POJOs if they are correctly annotated with the necessary annotations. For example, updating them, storing them in the database, etc. Hibernate entities or the hibernate persistence context are in charge of these POJOs.
The built-in Java annotations @Override, @SuppressWarnings, and @Deprecated are a few examples.
There are mainly 4 states of the Hibernate Lifecycle:
Transient State
Persistent State
Detached State
Removed State
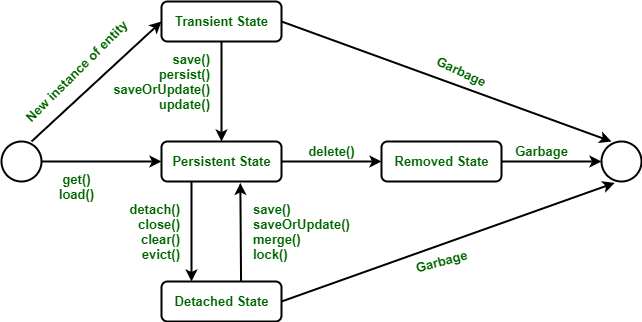
1.Transient State
The initial phase of an entity object is referred to as the transient state. When we create an instance of a Plain Old Java Object (POJO) class using the new operator, the object enters the transient state. During this stage, the object is not associated with any Hibernate session, meaning it is not linked to a database table. Consequently, modifications made to the data in the POJO class do not impact the corresponding database table, as the transient object operates independently of Hibernate and resides in the heap memory.
The transient state can manifest in two scenarios:
1. When objects are produced by an application but lack a connection to any session.
2. When objects are generated by a closed session.
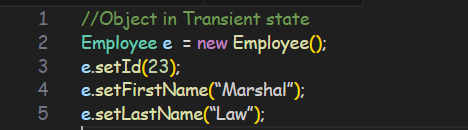
2.Persistent State
Once the object establishes a connection with the Hibernate Session, it transitions to the Persistent State. Consequently, there are two methods to convert the Transient State to the Persistent State:
1. By using the Hibernate session, store the entity object in the database table.
2. By using the Hibernate session, load the entity object into the database table.
In this state, each object corresponds to a single row in the database table. Thus, any modifications made to the data are promptly detected by Hibernate, leading to corresponding changes in the associated database table.
The following methods are provided for achieving the persistent state:
session.persist(e)
session.save(e)
session.saveOrUpdate(e)
session.update(e)
session.merge(e)
session.lock(e)

3.Detached State
To transition an object from the Persistent State to the Detached State, it is necessary to either close the session or clear its cache. Once the session is closed or the cache is cleared, any alterations to the data no longer impact the corresponding database table. The detached object can later be reconnected to a new Hibernate session as needed. To achieve this reconnection, the following methods can be employed:
merge()
update()
load()
refresh()
save()
update()
The subsequent methods are employed for managing the detached state:
session.detach(e);
session.evict(e);
session.clear();
session.close();
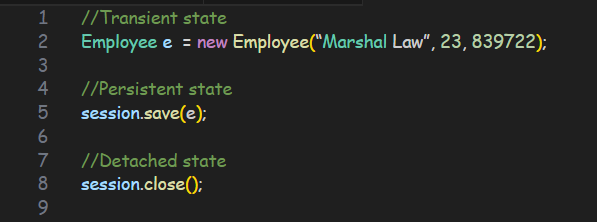
4.Removed State
In the Hibernate lifecycle, the removed state is the final stage. When an entity object is deleted from the database using the delete() operation, it enters the removed state. In this state, any modifications to the data will not impact the corresponding database table.
We can remove entity objects by calling session.delete().
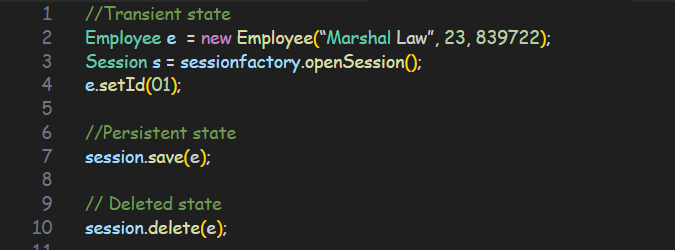
Comments