Introduction to Selenium WebDriver
- Kinjal Patel
- Aug 4, 2023
- 4 min read
WebDriver comes as an extended version of Selenium RC with superfluous advantages and addresses many of its limitations. WebDriver extends its support to many latest browsers and platforms, unlike Selenium IDE. WebDriver also doesn’t require the Selenium server to be started prior to the execution of the test scripts, unlike Selenium RC.
WebDriver directly calls the methods of different browsers hence we have separate drivers for each browser. Some of the most widely used web drivers include:
Mozilla Firefox Driver (Gecko Driver)
Google Chrome Driver
Internet Explorer Driver
Opera Driver
Safari Driver
HTML Unit Driver
Features of Selenium WebDriver
Browser Compatibility
Language Support
Speed
Drivers, Methods, and Classes
Selenium WebDriver- Architecture
There are four basic components of WebDriver Architecture:
Selenium Language Bindings
JSON Wire Protocol
Browser Drivers
Real Browsers
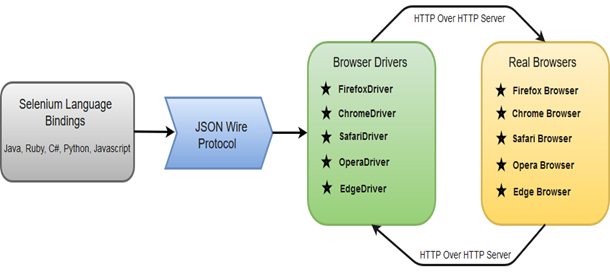
Selenium Language Bindings / Selenium Client Libraries
Selenium developers have built language bindings/Selenium Client Libraries in order to support multiple languages. For instance, if you want to use the browser driver in Java, use the Java bindings.
JSON Wire Protocol
JSON (JavaScript Object Notation) is an open standard for exchanging data on the web. It supports data structures like objects and arrays. So, it is easy to write and read data from JSON. JSON Wire Protocol provides a transport mechanism to transfer data between a server and a client. JSON Wire Protocol serves as an industry standard for various REST web services.
Browser Drivers
Selenium uses drivers, specific to each browser in order to establish a secure connection with the browser without revealing the internal logic of the browser's functionality. The browser driver is also specific to the language used for automation such as Java, C#, etc.
HTTP request is generated and sent to the browser driver for each Selenium command.
The driver receives the HTTP request through the HTTP server.
HTTP Server decides all the steps to perform instructions which are executed on the browser.
Execution status is sent back to HTTP Server which is subsequently sent back to automation script.
Selenium WebDriver- Locating Strategies
Just like Selenium IDE, WebDriver uses the same set of locating strategies for specifying the location of a particular web element.
Since we are using WebDriver with Java; each locating strategy has its own command in Java to locate the web elements.
Locating Strategies By ID
Locating Strategies By Name
Locating Strategies By Class Name
Locating Strategies By Tag Name
Locating Strategies By Link Text
Locating Strategies By Partial Link Text
Locating Strategies By CSS
Locating Strategies By XPath
Methods for locators
driver.findElement(By. name("lst-ib"))
driver.findElement(By.className (“”))
driver.findElement(By.tagName("input")).
driver.findElement(By.xpath("lst-ib"))
driver.findElement(By.linkText("lst-ib"))
driver.findElement(By.partialLinkText (<"This is">))
Selenium WebDriver- Commands
The commands provided by Selenium WebDriver can be broadly classified into the following categories:
Browser Commands
Navigation Commands
WebElement Commands
Selenium WebDriver - Browser Commands
1. Get Command
get(String arg0) : void
In WebDriver, this method loads a new web page in the existing browser window. It accepts String as parameter and returns void.
driver.get(URL);
2. Get Title Command
getTitle(): String
In WebDriver, this method fetches the title of the current web page. It accepts no parameter and returns a String.
String Title = driver.getTitle();
3. Get Current URL Command
getCurrentUrl(): String
In WebDriver, this method fetches the string representing the Current URL of the current web page. It accepts nothing as parameter and returns a String value.
String CurrentUrl = driver.getCurrentUrl();
4. Get Page Source Command
getPageSource(): String
In WebDriver, this method returns the source code of the current web page loaded on the current browser. It accepts nothing as parameter and returns a String value.
String PageSource = driver.getPageSource();
5. Close Command
close(): void
This method terminates the current browser window operating by WebDriver at the current time. If the current window is the only window operating by WebDriver, it terminates the browser as well. This method accepts nothing as parameter and returns void.
driver.close();
6. Quit Command
quit(): void
This method terminates all windows operating by WebDriver. It terminates all tabs as well as the browser itself. It accepts nothing as parameter and returns void.
driver.quit();
Selenium WebDriver - Navigation Commands
1. Navigate To Command
In WebDriver, this method loads a new web page in the existing browser window. It accepts String as parameter and returns void.
driver.navigate().to("www.stadsolution.com");
Note: same as get command
2. Forward Command
In WebDriver, this method enables the web browser to click on the forward button in the existing browser window. It neither accepts anything nor returns anything.
driver.navigate().forward();
3. Back Command
In WebDriver, this method enables the web browser to click on the back button in the existing browser window. It neither accepts anything nor returns anything.
driver.navigate().back();
4. Refresh Command
In WebDriver, this method refresh/reloads the current web page in the existing browser window. It neither accepts anything nor returns anything.
driver.navigate().refresh();
Selenium WebDriver - WebElement Commands
1. Clear Command
clear() : void
2. Sendkeys Command
sendKeys(CharSequence? KeysToSend) : void
driver.findElement(By.id("UserName")).sendKeys(“Selenium");
3. Click Command
click() : void
driver.findElement(By.linkText(“STAD Solution")).click();
4. IsDisplayed Command
isDisplayed() : boolean
boolean staus = driver.findElement(By.id("UserName")).isDisplayed();
5. IsEnabled Command
isEnabled() : boolean
boolean staus = driver.findElement(By.id("UserName")).isEnabled(); //Or can be used as
WebElement element = driver.findElement(By.id("userName"));
boolean status = element.isEnabled(); // Check that if the Text field is enabled, if yes enter value if(status){ element.sendKeys(“selenium); }
6. IsSelected Command
isSelected() : boolean
boolean staus = driver.findElement(By.id("Sex-Male")).isSelected();
7. Submit Command
submit() : void
8. GetText Command
getText() : String
WebElement element = driver.findElement(By.xpath("anyLink"));
String linkText = element.getText();
9. GetTagName Command
getTagName() : String
String tagName = driver.findElement(By.id("SubmitButton")).getTagNa me();
10. getCssValue Command
getCssvalue() : String
element.getCssValue();
11. getAttribute Command
getAttribute(String Name) : String
WebElement element = driver.findElement(By.id("SubmitButton"));
String attValue = element.getAttribute("id"); //This will return "SubmitButton"
12. getSize Command
getSize() : Dimension
WebElement element = driver.findElement(By.id("SubmitButton"));
Dimension dimensions = element.getSize();
System.out.println("Height :" + dimensions.height + "Width : "+ dimensions. width);
13. getLocation Command
getLocation() : Point
WebElement element = driver.findElement(By.id("SubmitButton"));
Point point = element.getLocation(); System.out.println("X cordinate : " + point.x + "Y cordinate: " + point.y);
Comments