"Java's Art of Adaptation: Exploring Polymorphism"
- Sukhpreet Kaur
- Mar 19, 2024
- 3 min read
The term polymorphism denotes the presence of multiple forms. Put simply, Java Polymorphism refers to the capability of a message to manifest in various forms. Throughout this discussion, we'll delve into the concept of polymorphism and explore its different types.
What does Polymorphism signify in Java?
Polymorphism stands out as a pivotal feature within Object-Oriented Programming. It empowers the execution of a singular action through diverse avenues. In essence, polymorphism enables the definition of a single interface with the capacity for multiple implementations. The term itself, stemming from "poly" meaning many and "morphs" signifying forms, encapsulates the idea of multifaceted expressions.
Types of Polymorphism in Java
In Java, polymorphism exhibits itself through two main forms: compile-time (or static) polymorphism and runtime (or dynamic) polymorphism.
Compile-Time(or static) Polymorphism:
Runtime(or dynamic) Polymorphism
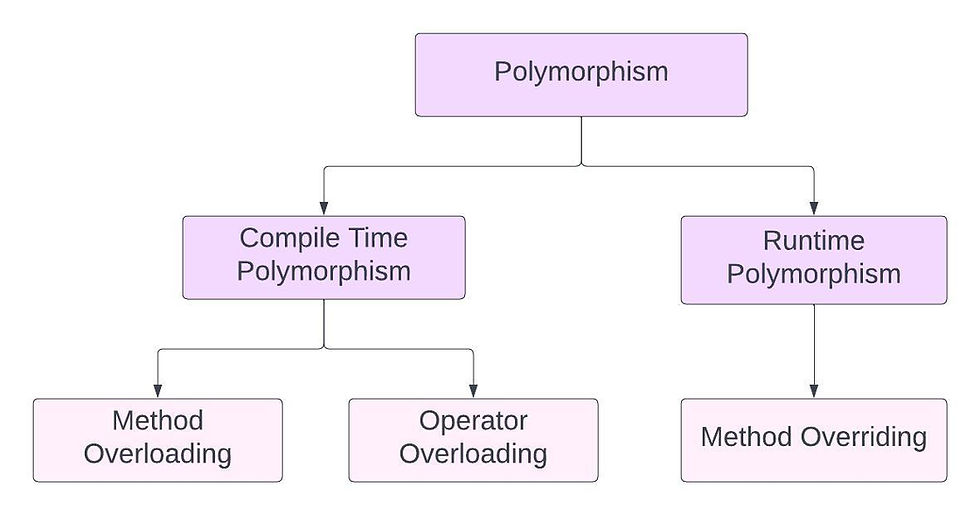
In this post, we'll explore Method Overloading and Method Overriding.
Method Overloading:
Method overloading in Java allows for the creation of multiple methods within a single class, all sharing the same name but featuring distinct parameter lists. This enables the implementation of methods that execute similar tasks but handle varying data types or numbers of parameters.
Method Overloading is a compile time polymorphism, as the decision is made by the compiler which method to invoke based on the method signature. It is a robust Java feature which enhances code readability and reusability by offering various approaches to execute analogous operations, all without needing distinct method names.
First Example of Method Overloading:
class Example {
void display(int num) {
System.out.println("Number: " + num);
}
void display(String str) {
System.out.println("String: " + str);
}
}
In this instance, the compiler determines which 'display' method to invoke based on the type of argument provided in the method calling.
Second Example of Method Overloading:
public class Example {
// Method to calculate the sum of two integers
public int sum(int a, int b) {
return a + b;
}
// Method to calculate the sum of three integers
public int sum(int a, int b, int c) {
return a + b + c;
}
}
public class Main {
public static void main(String args[]) {
System.out.println(Example.sum(2,4)); //Method with 2 arguments is called
System.out.println(Example.sum(3,4,5)); //Method with 3 arguments is called
}
}
In this instance, the compiler determines which 'sum' method to invoke based on the number of arguments provided in the method calling.
Method Overriding:
When a subclass (child class) defines a method with the same signature as declared in its parent class, it's termed as method overriding in Java. Put differently, method overriding occurs when a subclass furnishes a distinct implementation of a method declared by one of its parent classes.
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
// Override the sound() method of the superclass
void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String args[]) {
Animal myAnimal = new Dog(); // Dog object referred by Animal reference
myAnimal.sound(); // Calls the sound() method of Dog class
}
}
In this scenario, the Dog class inherits from the Animal class and provides its own version of the sound() method. Consequently, when myAnimal.sound() is invoked, it triggers the sound() method from the Dog class, resulting in the output "Dog barks" on the console. This runtime behavior is contingent upon the specific object being referenced.
Conclusion
In summary, Java polymorphism stands as a potent tool elevating code flexibility, extensibility, and maintainability. By employing method overloading and method overriding, Java empowers developers to craft adaptable code capable of accommodating diverse data types and scenarios, promoting code reuse and simplifying complex situations.
Polymorphism facilitates dynamic method binding during runtime, enabling uniform treatment of objects across varying types via inheritance and interfaces. This versatility not only enhances code readability and conciseness but also streamlines the development of modular and scalable software solutions.
Thank you for taking the time to read this blog post.
Happy Learning !!
Comentários