Serialization and Deserialization
- arshpalsingh0912
- Oct 10, 2023
- 4 min read
In this blog, we will be learning about the concept of serialization and deserialization. We will be covering serialization, deserialization, transient keyword, static vs transient keyword, final vs transient keyword. Moreover, we will try to cover Objectgraph and customized serialization.
Serialization :
In simple words, serialization is nothing but process of writing state of object to a file.
But, strictly speaking, it is process of converting an object from java supported form to either File supported form or network supported form. Here, the object is converted into byte stream and then saved to file.
We can achieve serialization using FileOutputStream and ObjectOutputStream.
FileOutputStream is a class in java which is available in java.io package and is used for writing the binary data to file.
ObjectOutputStream is a class in java which is available in java.io package and is used for serializing objects as it allows you to write the object to output stream(here it is FileOutputStream) and later it can be serialized using ObjectInputStream.
This is the flow of serialization using the diagram :

Deserialization :
In simple words, deserialization is nothing but process of reading state of an object from a file. But, strictly speaking, it is the process of converting an object from either a file or network supported form into java supported form. Here, the object is reconstructed from byte stream from file.
We can achieve deserialization using FileInputStream and ObjectInputStream.
FileInputStream is a class in java which is available in java.io package and is used for reading the binary data from file.
ObjectInputStream is a class in java which is available in java.io package and is used for reading objects from input stream(here, it is FileInputStream) particularly when those objects are already been serialized.
This is the flow of deserialization using the diagram :
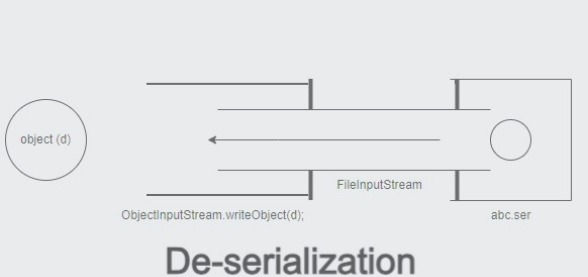
Let us understand concept of serialization and deserialization with code :
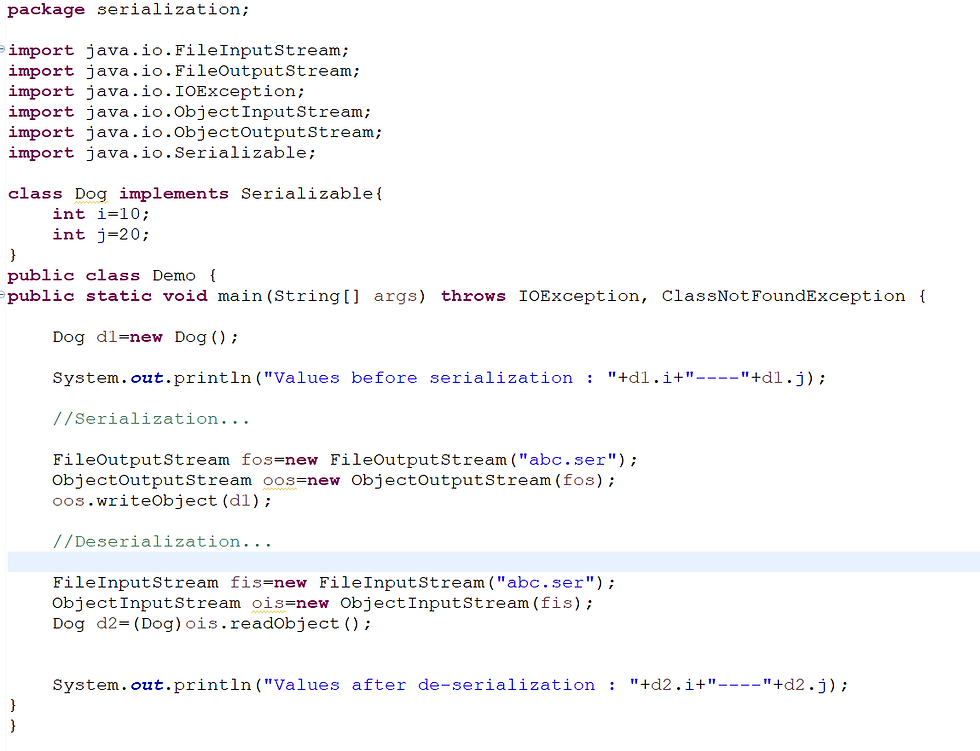
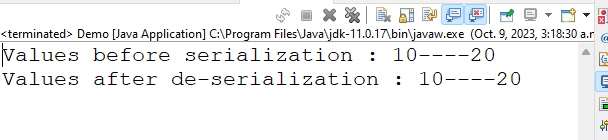
Important points to remember :
we can serialize only the serializable objects and the object is only said to be serializable if and only if the corresponding class implements serializable interface.
Serializable Interface is present in java.io package and it is a marker interface as it does not contain any method in it.
Transient keyword:
Transient is modifier which is applicable only for variables.
At the time of serialization if we do not want to save the value of a particular variable to meet security constraints, then we should go for transient keyword.
At the time of serialization, JVM ignores the original value of transient variables and saves the default value to the file.
We can remember the transient keyword as NOT TO SERIALIZE.
Let us understand transient keyword with help of code :
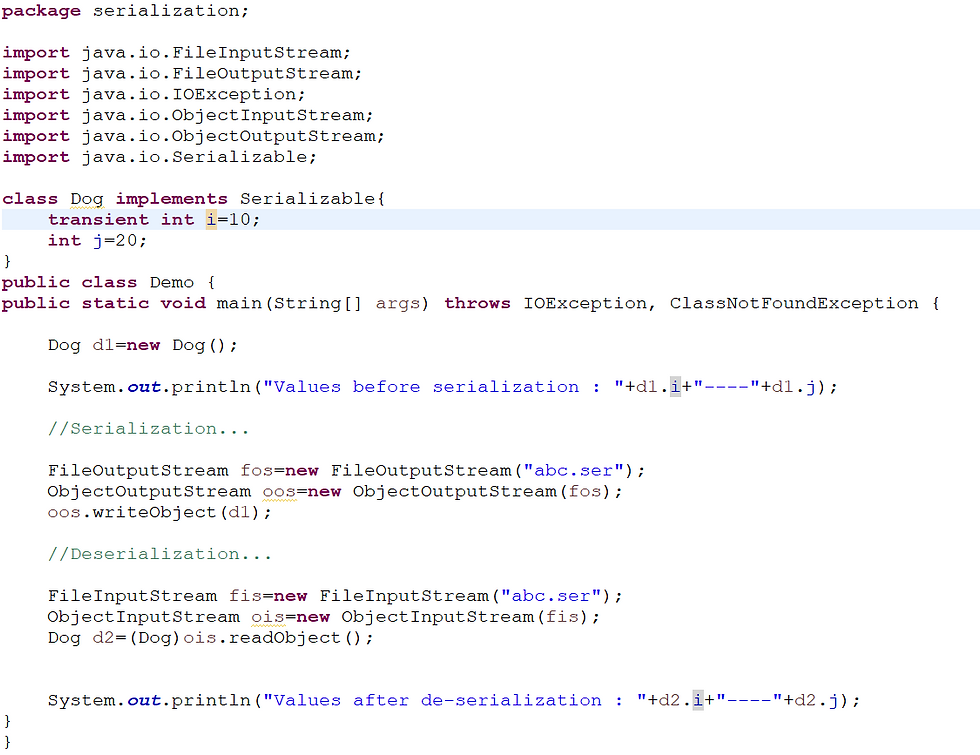

In the above example as we have declared the variable i as transient , so it will not take part in serialization, moreover it will store the default value of i variable which is 0 for int datatype.
Static vs transient keyword :
Static Variables are not part of the Object state hence they do not take part in serialization.
Due to this, declaring a static variable as transient, there is no use or impact.
Let us understand this with help of code :
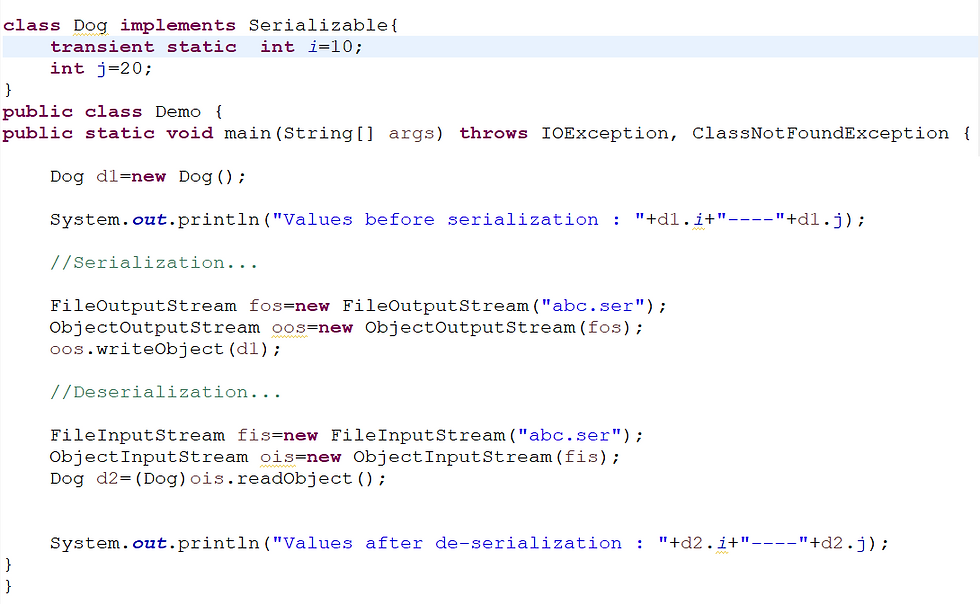

Final vs transient keyword :
Final variable participates in serialization directly by their values which it gets at the time of compilation by JVM.
So, even if we are declaring the final variable as the transient there is no use or impact.
Let us understand this with help of code :
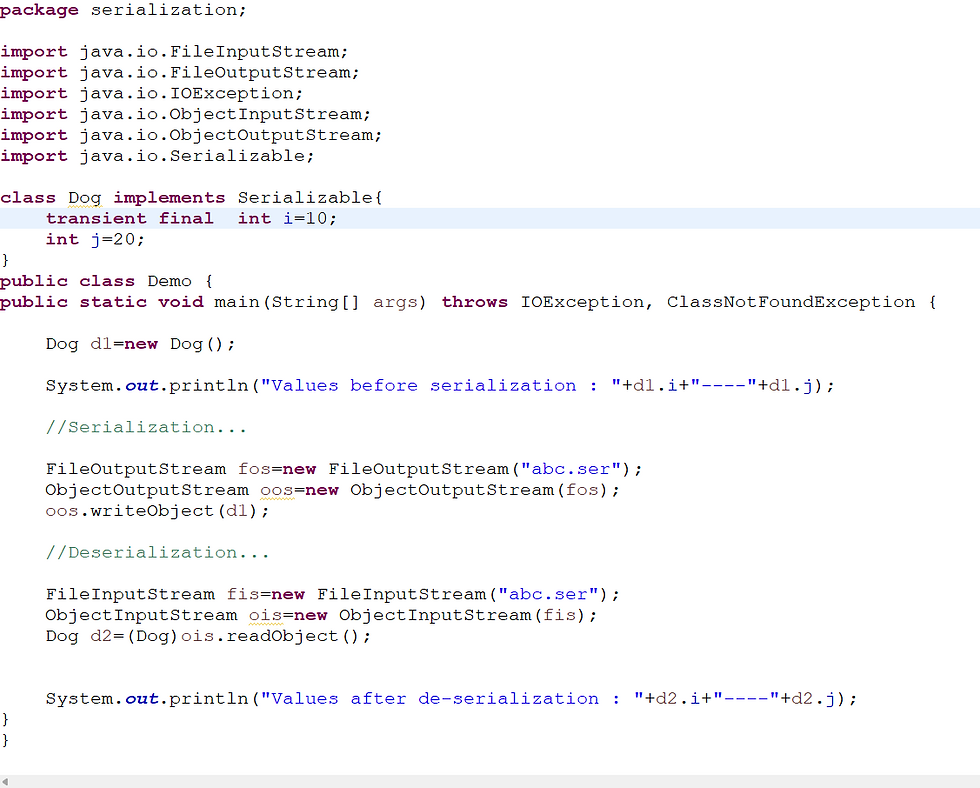

NOTE :
We can serialize any number of objects to the file , but the order in which we serialized is important as we have to deserialize in the same order.
In simple words, we can say that order of serialization is important.
Let us understand this concept with help of example :
Here, we are following the Order which is same for serialization and deserialization :
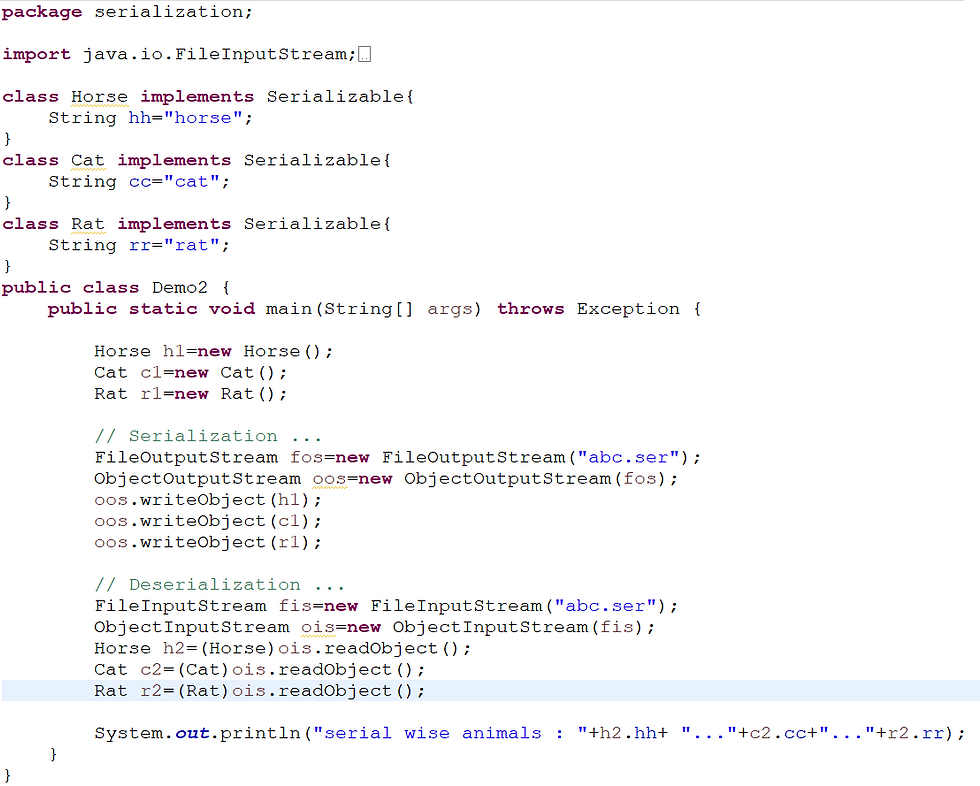

2. In this example, we will be getting the ClassCastException if we do not follow the same order for serialization and deserialization :
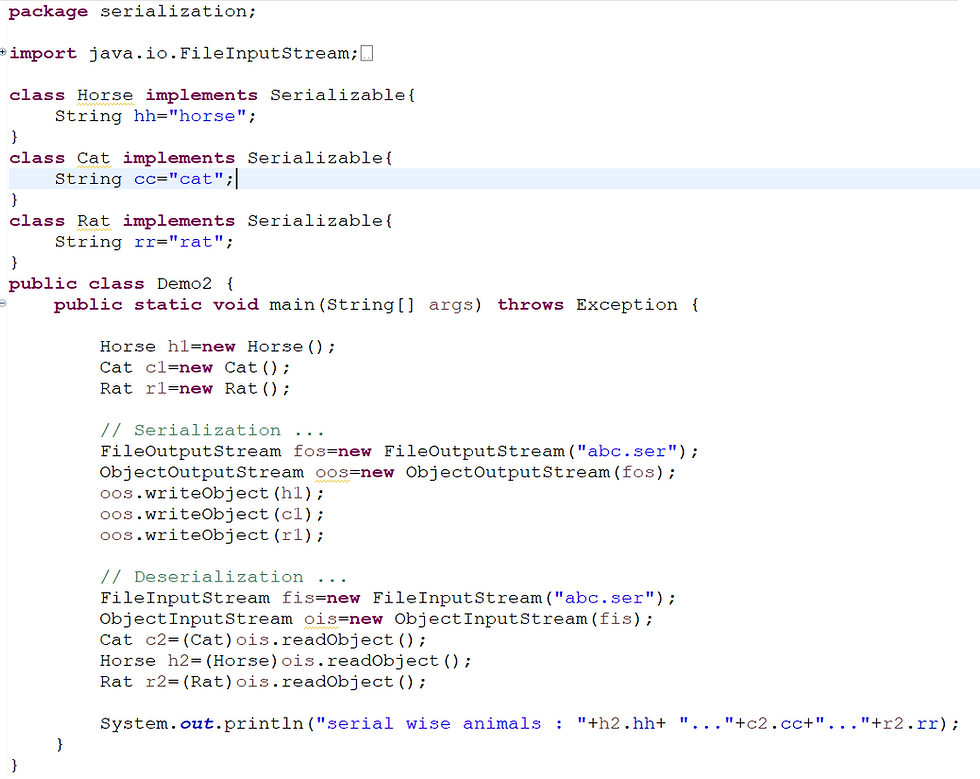

Object Graph :
Whenever we are serializing an object, the set of all objects which are reachable from that object will be serialized automatically. This group of objects is nothing but Object graph.
In Object Graph, every object should be serializable. If at least one object is non-serializable then we will get Runtime Exception saying NotSerializable.
Let us understand this concept with help of example :
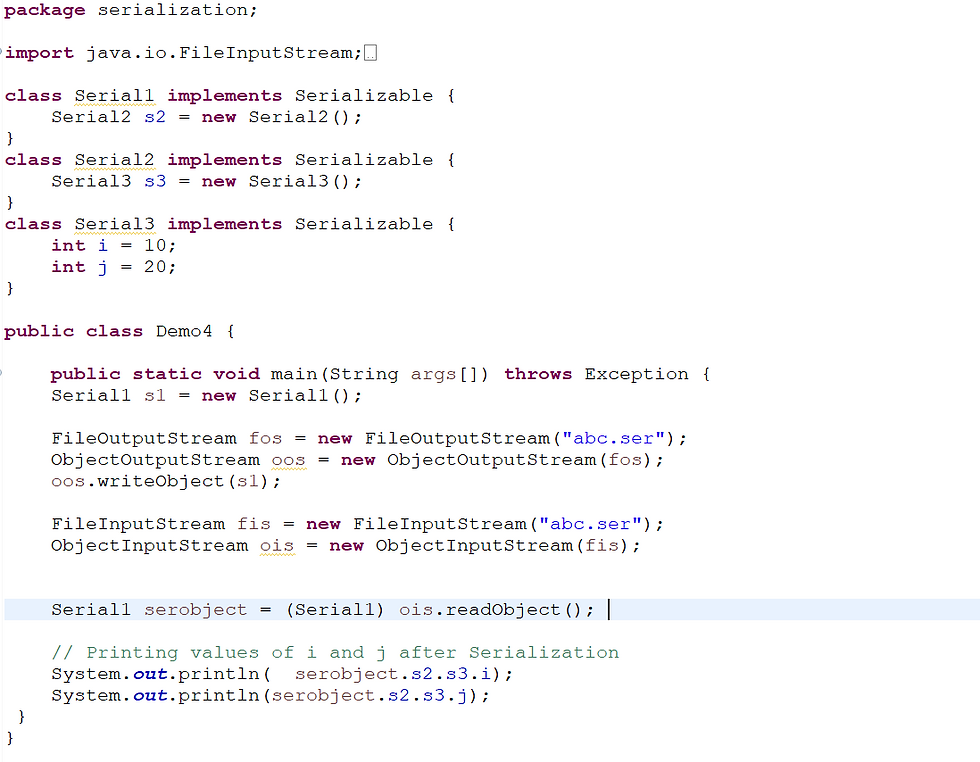
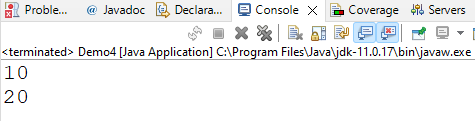
Diagrammatic view for code :
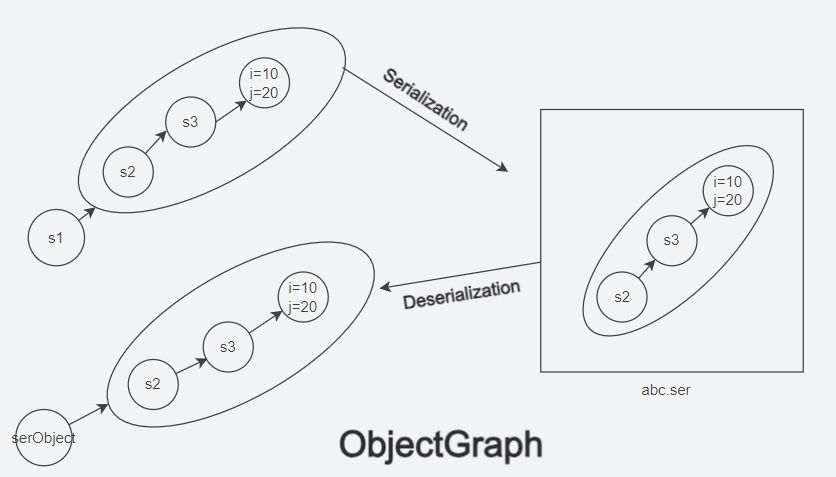
Customized Serialization :
Customized serialization refers to the process of defining how the object or data structure should be converted into a format that can be easily stored, transmitted and reconstructed and then implementing this conversion logic according to specific requirement.
Need of Customized Serialization :
The need for customized serialization arises when the default serialization mechanism are insufficient for particular use case. In default serialization, there may be loss of information because of transient keyword.
Example showing problems occurring because of default serialization :
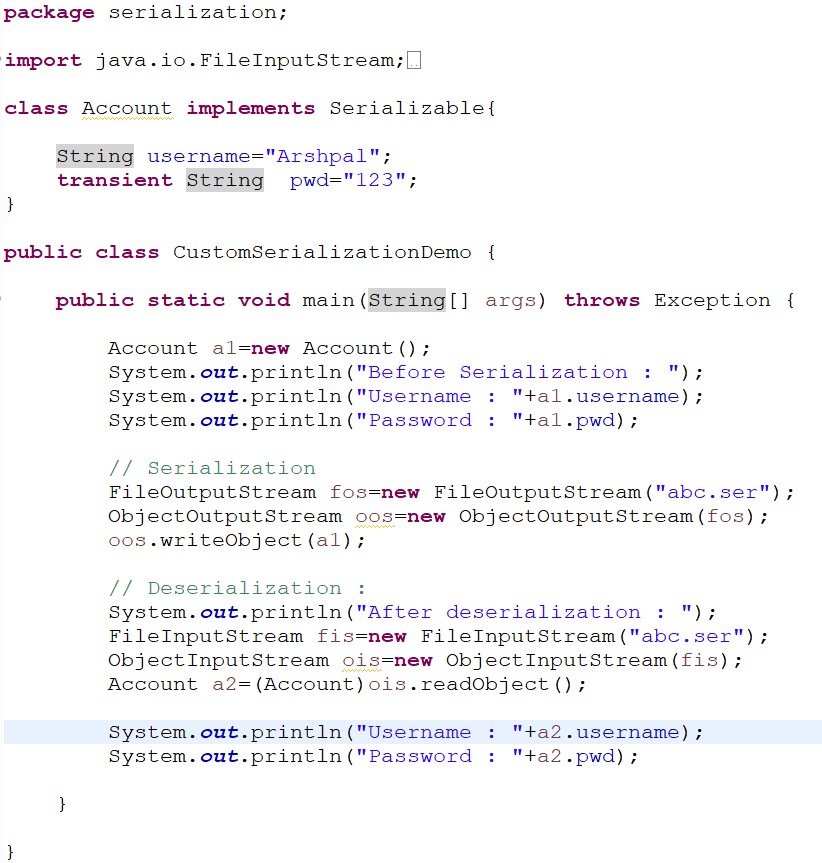
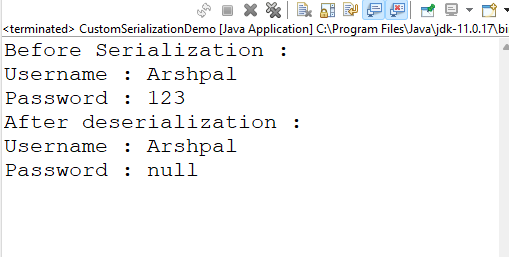
You can clearly see in the above example that because of the using the transient keyword we are getting password as null after deserialization. Hence, there is loss of information due to transient keyword.
To solve this we are using customized serialization.
Methods used for implementing customized serialization :
private void writeObject(ObjectOutputStream oos) throws Exception
This method will be executed automatically at time of serialization. Hence, while performing serialization, if we want to do any extra work we to write corresponding code in this method only.
2. private void readObject(ObjectInputStream ois) throws Exception
This method will be executed automatically at time of de-serialization. Hence, if we want to do any extra work, we have to define the corresponding code in this method only.
The above methods are callbacks methods because these methods will be executed automatically by JVM. We have to define these methods in the class where we have to do the extra work for serialization.
Let us understand this with help of example :
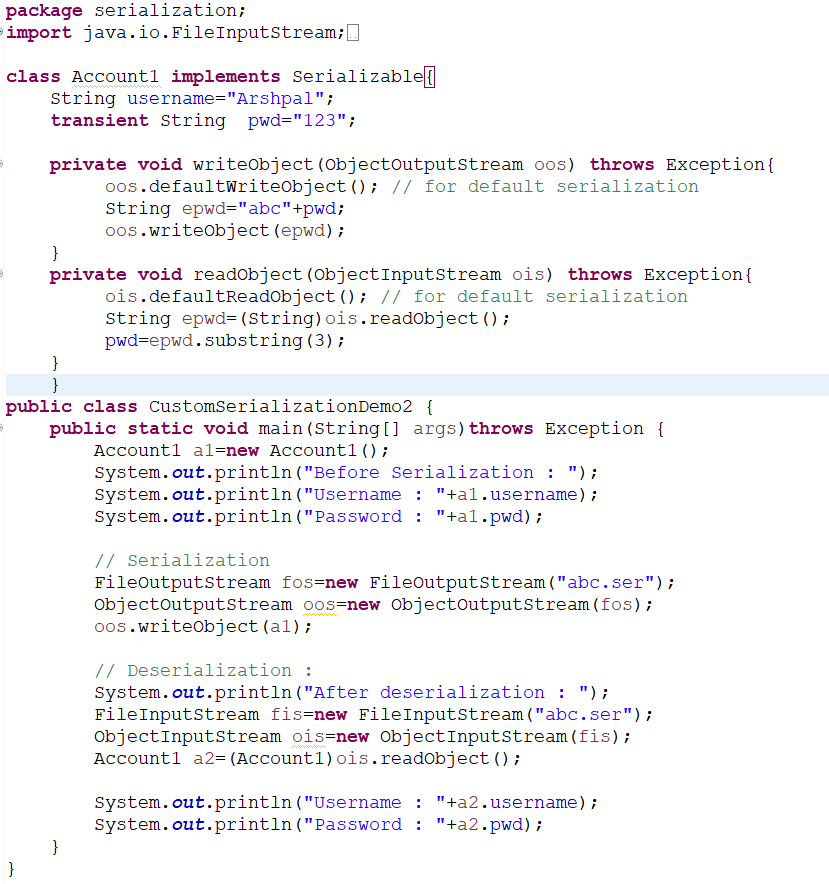
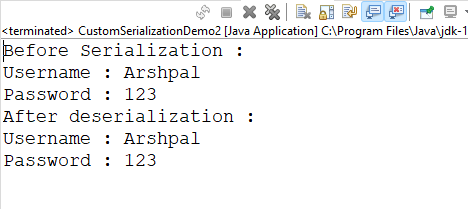
You can see in the above example that even after declaring the variable as transient , we are able to serialize it and then deserialize it as well. This is only possible due to customized serialization.
Comments