Servlet is a server-side Java program module that handles client requests and implements the servlet interface. Servlets can respond to any type of request, and they are commonly used to extend the applications hosted by web servers.
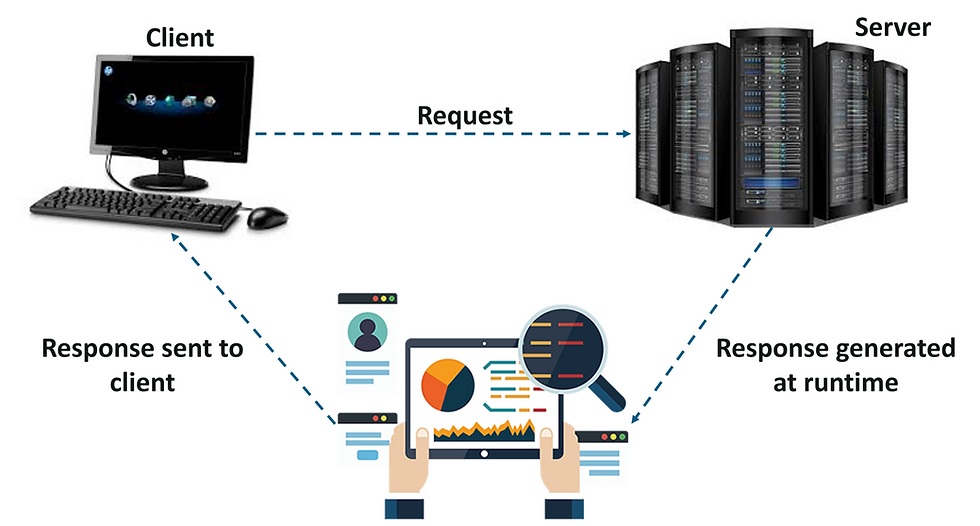
In order to create a servlet, we need to follow a few steps in order. They are as follows:
Create a directory structure
Create a Servlet
Compile the Servlet
Add mappings to the web.xml file
Start the server and deploy the project
Access the servlet
Now, let’s take an example where I will be creating a simple login servlet using HTTP Servlet and display the output in the browser.
First, I will create index.html file
<!DOCTYPE html>
<html>
<body>
<form action="Login" method="post">
<table>
<tr>
<td>UserName:</td>
<td><input type="text" name="userName"></td>
</tr>
<tr>
<td>UserPassword:</td>
<td><input type="password" name="userPassword"></td>
</tr>
</table>
<input type="submit" value="Login">
</form>
</body>
</html>
Let's create a database connection file.
package com.miit.ServletDemo;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
static Connection connection;
public static Connection initializeDatabase() throws SQLException, ClassNotFoundException {
Class.forName("com.mysql.cj.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:5080/java_sep","root","root");
return connection;
}
}
Now create a Java file.
package com.miit.ServletDemo;
import java.io.IOException;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/LoginServlet")
public class LoginServlet extends HttpServlet{
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
try {
String username = req.getParameter("username");
String password = req.getParameter("password");
PreparedStatement ps = DatabaseConnection.initializeDatabase().prepareStatement("select * from registration where User_Name = ? and User_Password = ?");
ps.setString(1, username);
ps.setString(2, password);
boolean status = ps.execute();
if(status==true) {
req.getRequestDispatcher("/home.jsp").include(req, resp);
}
else {
resp.getWriter().write("Wrong UserName or Password... Try again...");
}
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
The web.xml file will be present in the WEB-INF folder of your web content. Once you get your web.xml file ready, you need to add the mappings to it. Let’s see how mapping is done using the below example:
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd">
<web-app>
<display-name>Archetype Created Web Application</display-name>
<servlet>
<servlet-name>Login</servlet-name>
<servlet-class>com.miit.ServletDemo</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Login</servlet-name>
<url-pattern>/LoginServlet</url-pattern>
</servlet-mapping>
</web-app>
So, this is how a servlet is created and configured. Now you can run this program as Run On Server.
Comments