Any programmer when he/she wants to represent a sequence of characters String is used. When programming, Strings are one of the most used classes. But Strings are immutable i.e. can not be modified. To overcome this situation String Buffer and StringBuilder were introduced in Java. In this article, we will discuss in detail about concepts of String Buffer and StringBuilder. Before that, we will also summarize concepts of String in Java for better understanding.
Strings:
Strings are used to store sequences of characters in Java. Any operation that appears to modify a string creates a new Object. The basic syntax to write strings in Java is as follows:
String string = “Hello Java”;
Strings are immutable, which means we cannot change or modify the string.
String Buffer:
String Buffer is similar to string but allows for modification. It has various methods to edit, append, and delete strings. The usage of String Buffer by many threads is secure. No more than one thread can simultaneously call a String Buffer method. String Buffer has four constructors as follows:
String Buffer (): This creates an empty string buffer with 16 characters capacity.
String Buffer (CharSequence chars): It creates a String Buffer containing the same characters as CharSequence.
String Buffer (int size): It creates a String Buffer with no characters with a specified initial capacity.
String Buffer (String str): It creates a String Buffer object with the initial specified string.
Some of the methods to modify strings via String Buffer are Length (), capacity (), append (), Insert (), char At (), and many more.
Please consider following example for syntax of String Buffer:
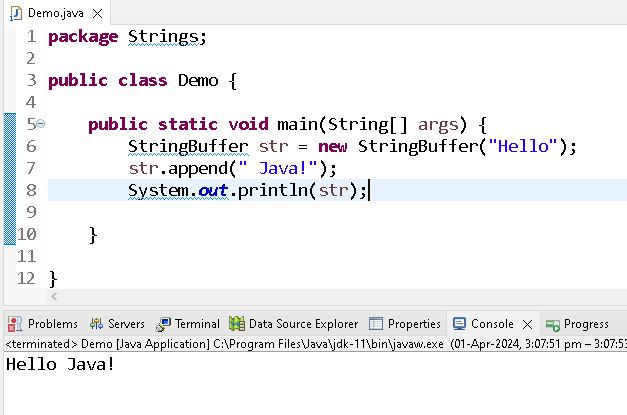
String Buffer:
Similar to String Buffer, the StringBuilder in Java represents a mutable sequence of characters. It also allows efficient string manipulation, such as appending, inserting, or modifying characters within the string, without creating new objects. But where these two differ is in the synchronization. StringBuilder is not synchronized which means it is not thread-safe.
String Builder has also the same four defined constructors in Java as String Buffer which are String Builder (), StringBuilder(int size), StringBuilder(CharSequence seq), and StringBuilder(String str).
Some of the methods to modify strings via String Buffer are append (), replace (), Substring (), and many more.
Please consider the following example for the syntax of String Builder:

Difference Between String Buffer() And StringBuilder:
String Buffer Class | StringBuilder Class |
String Buffer has already been present since Java was introduced. | StringBuilder was introduced in Java 5 |
String Buffer is synchronized, which means multiple threads cannot call String Buffer methods simultaneously. | StringBuilder is asynchronized. Multiple threads can call its methods simultaneously. |
String Buffer is a thread-safe class. | StringBuilder is not thread thread-safe class. |
String Buffer is slower because of the above two characteristics. | StringBuilder serves faster performance in terms of speed and efficiency. |
Commentaires