We can implement dependency injection with following:
i) Constructor-based injection.
ii) Setter-based injection.
iii) Field-based injection.
i)Constructor Injection:
In constructor injection, the dependencies required for the class are provided as arguments to the constructor.
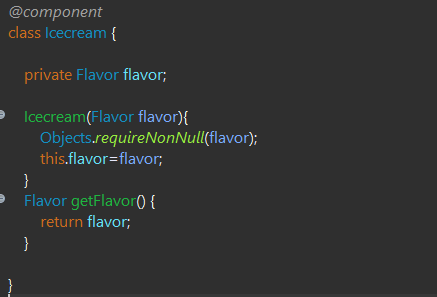
Before Spring 4.3, we had to add an @Autowired annotation to the constructor. With newer versions, this is optional if the class has only one constructor.
In the Cake class above, since we have only one constructor, we don’t have to specify the @Autowired annotation.
When we have a class with multiple constructors, we need to explicitly add the @Autowired annotation to any one of the constructors so that Spring knows which constructor to use to inject the dependencies.
ii) Setter Injection:
In setter-based injection, we provide the required dependencies as field parameters to the class and the values are set using the setter methods of the properties. We have to annotate the setter method with the @Autowired annotation.
The Pizza class requires an object of type Topping. The Topping object is provided as an argument in the setter method of that property:

Spring will find the @Autowired annotation and call the setter to inject the dependency.
iii) Field Injection:
With field-based injection, Spring assigns the required dependencies directly to the fields on annotating with @Autowired annotation.
In this example, we let Spring inject the Topping dependency via field injection:
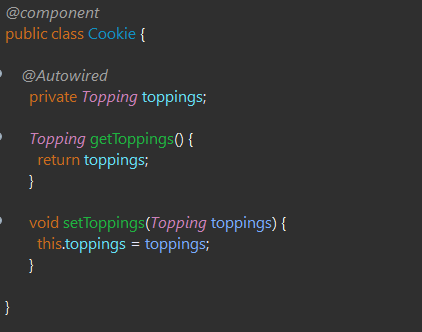
*Why is Constructor Injection recommended?
We create an object by calling a constructor. If the constructor expects all required dependencies as parameters, then we can be 100% sure that the class will never be instantiated without its dependencies injected.
The IoC container makes sure that all the arguments provided in the constructor are available before passing them into the constructor. This helps in preventing the infamous NullPointerException.
Constructor injection is extremely useful since we do not have to write separate business logic everywhere to check if all the required dependencies are loaded, thus simplifying code complexity.
Bình luận