Unlocking the Power of Java Stream API
- ankurpatel2608
- Oct 10, 2023
- 3 min read
Introduction
In the ever-evolving landscape of programming languages, Java remains a cornerstone. With each new release, it continues to adapt and provide developers with more efficient tools for handling complex tasks. Java 8 brought with it a transformative feature that changed the way developers work with collections: the Stream API. In this comprehensive guide, we will explore the Java Stream API in depth, covering its core concepts, advanced features, and practical use cases.
Understanding the Java Stream API
The Java Stream API is a robust abstraction for processing sequences of data in a functional and declarative style. It enables developers to work with collections, such as lists, arrays, or sets, in a more expressive and efficient manner. Java Streams are not only powerful but also flexible, allowing you to perform operations on data similar to SQL queries or functional programming constructs.
Key Concepts
Before we dive into practical examples, let's grasp the fundamental concepts of the Java Stream API:
Stream: A stream is a sequence of data elements that can be processed either sequentially or in parallel. It's not a data structure but rather a way to access and manipulate data from a source.
Intermediate Operations: Intermediate operations transform a stream into another stream. These operations include filter, map, distinct, and more. They are lazy, meaning they are not executed until a terminal operation is invoked.
Terminal Operations: Terminal operations produce a result or a side-effect. Examples include forEach, reduce, and collect. Terminal operations trigger the execution of the stream pipeline.
Parallel Streams: Java Streams can be executed in parallel, taking advantage of multi-core processors for enhanced performance. Parallel streams can be created with the parallel() method.
Practical Usage
Now, let's explore practical scenarios where the Java Stream API shines:
Filtering Data: The filter operation allows you to selectively include elements in a stream based on a condition. For instance, filtering even numbers from a list:

This code filters out odd numbers, leaving only even numbers in the evenNumbers list.
Mapping Data: The map operation transforms elements in a stream. Consider converting a list of strings to uppercase:

Here, we've mapped all the names to uppercase strings, creating a new list of upperCaseNames.
Reducing Data: The reduce operation combines elements of a stream into a single result. Calculate the sum of a list of integers:

This code reduces the list of integers to a single sum.
Collecting Data: Use the collect operation to accumulate elements into a collection or another data structure. Collect a stream of objects into a map:

This code collects a list of Person objects into a map, where names are keys, and ages are values.
Parallel Processing: To leverage parallel processing, convert a sequential stream into a parallel stream using the parallel() method:

By simply changing .stream() to .parallelStream(), you can harness the power of multi-core processors for faster computation.
Advanced Concepts
Let's explore some advanced concepts related to the Java Stream API:
Lazy Evaluation
The Stream API employs lazy evaluation. Intermediate operations are not executed immediately; instead, they build a pipeline of operations. Execution occurs only when a terminal operation is invoked. This approach optimizes processing, especially with large datasets, by avoiding unnecessary computations.
Optional and Stream
Java Streams work seamlessly with Optional. When finding a single element that meets a condition, you can use findFirst() or findAny() in combination with filter. These methods return an Optional, allowing you to gracefully handle presence or absence of a value.
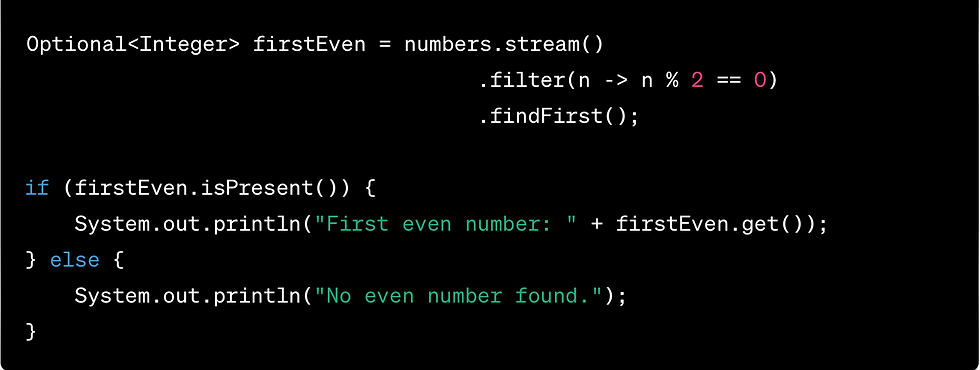
Stream Creation
Streams can be created from various sources:
From a List or Collection.
From an Array.
From a range of numbers using IntStream.
From a file using Files.lines() (requires error handling).
Each source allows you to work with data in a stream-oriented manner.
Conclusion
The Java Stream API is a game-changer for developers, offering a more functional, concise, and expressive way to manipulate collections of data. Whether you're filtering, mapping, reducing, collecting, or leveraging parallelism, the Stream API simplifies the process, resulting in cleaner, more maintainable code and improved performance. Embrace the Java Stream API, and elevate your Java programming skills to new heights.
Comments